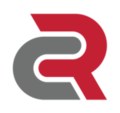 |
LibMultiSense
LibMultiSense Documentation
|
Go to the documentation of this file.
50 #include <opencv2/core/mat.hpp>
53 #if !defined(MULTISENSE_API)
54 #if defined (_MSC_VER)
55 #define MULTISENSE_API __declspec(dllexport)
57 #define MULTISENSE_API
65 using TimeT = std::chrono::time_point<std::chrono::system_clock, std::chrono::nanoseconds>;
130 std::array<std::array<float, 3>, 3>
K{{{0.0f, 0.0f, 0.0f}, {0.0f, 0.0f, 0.0f}, {0.0f, 0.0f, 0.0f}}};
136 std::array<std::array<float, 3>, 3>
R{{{0.0f, 0.0f, 0.0f}, {0.0f, 0.0f, 0.0f}, {0.0f, 0.0f, 0.0f}}};
142 std::array<std::array<float, 4>, 3>
P{{{0.0f, 0.0f, 0.0f, 0.0f}, {0.0f, 0.0f, 0.0f, 0.0f}, {0.0f, 0.0f, 0.0f, 0.0f}}};
152 std::vector<float>
D = {};
160 return std::array<float, 3>{(
P[0][0] == 0.0f ? 0.0f :
P[0][3] /
P[0][0]),
161 (
P[1][1] == 0.0f ? 0.0f :
P[1][3] /
P[1][1]),
181 std::optional<CameraCalibration>
aux = std::nullopt;
206 std::shared_ptr<const std::vector<uint8_t>>
raw_data =
nullptr;
260 template <
typename T>
261 std::optional<T>
at(
int w,
int h)
const
277 const size_t offset =
sizeof(T) * ((
width * h) + w);
286 cv::Mat cv_mat()
const;
315 auto it =
images.find(source);
318 throw std::runtime_error(
"No image found for requested DataSource");
984 std::optional<InternalConfig>
internal = std::nullopt;
990 std::optional<ExternalConfig>
external = std::nullopt;
1219 return input_camera_time + std::chrono::duration_cast<std::chrono::system_clock::duration>(
offset_to_host());
1231 std::optional<PtpStatus>
ptp = std::nullopt;
1246 std::optional<PowerStatus>
power = std::nullopt;
1256 std::optional<TimeStatus>
time = std::nullopt;
std::vector< ImuRate > rates
The available rates supported by this operating mode.
std::optional< ManualExposureConfig > manual_exposure
The exposure config to use if auto exposure is disabled.
ImagerType imager_type
The type of the imager.
@ RIGHT_RECTIFIED_COMPRESSED
float sharpening_percentage
The percentage strength of the sharpening gain to apply to the aux image Valid range is [0,...
float intensity
Lighting brightness ranging from 0 to 100.0.
uint64_t hardware_version
ID for the version of hardware.
std::string ip_address
The ip address of the camera (i.e.
A collection of IMU samples from the camera.
PixelFormat format
The format of the image data stored in the raw_data stored in the raw_data buffer.
std::vector< ImuSample > samples
A batched collection of IMU samples.
bool packet_delay_enabled
Add a small delay between the transmission of each packet to hopefully interact better with slower cl...
std::optional< CameraCalibration > aux
Calibration information for the aux camera (optional 3rd center camera)
float input_current
The current input current in Amperes.
A frame containing multiple images (indexed by DataSource).
const Image & get_image(const DataSource &source) const
Retrieve image by DataSource.
std::optional< OperatingMode > accelerometer
Configuration for the onboard accelerometer.
bool operator<(const Version &other) const
Convenience operator for comparing versions.
bool operator==(const AutoWhiteBalanceConfig &rhs) const
Equality operator.
StereoConfig stereo_config
The stereo configuration to use.
@ EXTERNAL
Drive lights via an external output.
uint32_t height
The height of the output image in pixels.
float max_gain
The auto exposure algorithm adjusts both exposure and gain.
bool operator==(const ImuRange &rhs) const
Equality operator.
Convenience wrapper for a version number See https://semver.org/.
std::string camera_name
The name of the MultiSense variant.
Config for the IMU sensor.
CameraCalibration left
Calibration information for the left camera.
uint32_t number_of_lights
The number of lights the MultiSense controls.
AutoExposureRoiConfig roi
The auto exposure region-of-interest used to restrict the portion of the image which the auto exposur...
std::string gateway
The gateway of the camera (i.e.
@ INTERNAL
Lights driven internally.
std::string device
The name of the IMU chip.
float frames_per_second
The target framerate the MultiSense should operate at.
DistortionType distortion_type
The type of the distortion model used for the unrectified camera.
std::vector< float > D
Coefficients for the distortion model.
uint32_t width
The width of the output image in pixels.
uint32_t decay
The decay rate used for auto-white-balance Valid range [0, 20].
std::optional< Measurement > gyroscope
The rotational velocity in degrees-per-second.
float red
The manual red white-balance setting Valid range is [0.25, 4].
float nominal_relative_aperture
The nominal relative aperture for the primary camera modules in f-stop.
float nominal_focal_length
The nominal focal length for the primary lens in meters.
Image specific configuration.
float gain
The desired electrical and digital gain used to brighten the image.
uint32_t pulses_per_exposure
The number of pulses of the light per single exposure.
The network configuration for the MultiSense.
HardwareRevision hardware_revision
The hardware revision of the MultiSense.
bool auto_white_balance_enabled
Enable or disable auto white balance.
Static status info for the MultiSense.
std::vector< DataSource > supported_sources
Data sources supported at that mode.
float target_intensity
The auto-exposure algorithm in digital imaging endeavors to achieve a specified target intensity,...
std::chrono::time_point< std::chrono::system_clock, std::chrono::nanoseconds > TimeT
A valid operating mode for the MultiSense.
bool operator==(const ExternalConfig &rhs) const
Equality operator.
TimeT ptp_frame_time
The MultiSense ptp timestamp associated with the frame.
float nominal_stereo_baseline
The nominal stereo baseline in meters.
Auto-exposure specific configuration.
CameraCalibration right
Calibration information for the right camera.
std::vector< SupportedOperatingMode > operating_modes
Supported operating modes.
uint8_t sharpening_limit
The maximum difference in pixels that sharpening is is allowed to change between neighboring pixels.
ColorImageEncoding aux_color_encoding
The encoding of the aux color image(s) in the frame.
PixelFormat
Pixel formats.
float right_imager_temperature
Temperature of the right imager in Celsius.
void add_image(const Image &image)
Add an image to the frame, keyed by the image's DataSource.
@ LEFT_DISPARITY_COMPRESSED
uint32_t decay
The desired auto-exposure decay rate.
bool operator==(const MultiSenseConfig &rhs) const
Equality operator.
float sample_rate
The sample rate for the sensor in Hz.
bool operator==(const NetworkTransmissionConfig &rhs) const
Equality operator.
std::string name
The name of the PCB This value can store at most 32 characters.
constexpr bool has_aux_camera() const
Determine if the MultiSense has a Aux color camera based on the DeviceInfo.
std::string netmask
The netmask of the camera (i.e.
std::optional< AuxConfig > aux_config
The image configuration to use for the aux camera if present.
Consolidated status information which can be queried on demand from the MultiSense.
std::chrono::microseconds startup_time
The time it takes for the light to reach full brightness.
Config for time-based controls.
std::optional< Source > gyroscope
Configuration specific to the gyroscope.
bool operator==(const AutoExposureRoiConfig &rhs) const
Equality operator.
std::string to_string() const
Convert a Version info to string for convenience.
size_t received_messages
The total number of valid messages received from the client.
float input_voltage
The current input voltage in volts.
bool operator==(const AutoExposureConfig &rhs) const
Equality operator.
std::chrono::nanoseconds offset_to_host() const
Compute the time offset which can manually be added to all camera timestamps to change the camera tim...
MaxDisparities
Predefined disparity pixel search windows.
Lighting config for lights integrated into the MultiSense.
std::vector< ImuRange > ranges
The available ranges supported by this operating mode.
@ OUTPUT_TRIGGER
A GPIO line is used to trigger an external light.
float threshold
The auto white balance threshold Valid range [0.0, 1.0].
float resolution
The min resolution the sensor can return.
std::string firmware_build_date
The date the firmware running on the camera was built.
std::optional< ImuConfig > imu_config
The imu configuration to use for the camera.
std::optional< Source > magnetometer
Configuration specific to the magnetometer.
std::chrono::microseconds max_exposure_time
The max exposure time auto exposure algorithm can set in microseconds Valid range is [0,...
A single IMU sample from the camera.
float power_supply_temperature
Temperature of the internal switching power supply in Celsius.
DataSource
Identifies which camera or data source the image is from.
CameraCalibration calibration
The scaled calibration associated with the image.
bool auto_exposure_enabled
Enable or disable auto exposure.
std::string imager_name
The name of the imager used by the primary camera.
Auto white balance specific configuration.
ImageConfig image_config
The image configuration to use for the main stereo pair.
Info about the available IMU configurations.
LensType lens_type
The type of the primary imager.
uint32_t revision
The revision number of the PCB.
std::array< float, 3 > rectified_translation() const
Get the translation vector in meters which translates points in the current CameraCalibration frame t...
MultiSenseInfo::Version firmware_version
The version of the firmware running on the camera.
A sample rate, and what impact it has on bandwidth.
int width
Width of the image in pixels.
bool flash
Enable flashing of the light.
NetworkInfo network
The network configuration of the MultiSense.
MaxDisparities disparities
The max number of pixels the MultiSense searches when computing the disparity output.
A generic measurement for a 3-axis IMU.
std::optional< NetworkTransmissionConfig > network_config
Config to control network transmission settings.
ImuRate rate
The specific IMU rate configuration specified in ImuInfo::Source table.
uint32_t imager_width
The native width of the primary imager.
HardwareRevision
MultiSense Hardware revisions.
std::optional< TimeConfig > time_config
Config for the MultiSense time-sync options.
std::string serial_number
The unique serial number of the MultiSense This value can store at most 32 characters.
Image specific configuration for the Aux imager.
std::optional< ManualWhiteBalanceConfig > manual_white_balance
The white balance parameters to use if auto white balance is disabled.
LightingType
MultiSense lighting types.
std::optional< T > at(int w, int h) const
Get a pixel at a certain width/height location in the image.
float bandwith_cutoff
The bandwith cutoff for a given IMU mode in Hz.
std::chrono::nanoseconds client_host_time
The time of the host machine running the client when the status request was sent.
CameraStatus camera
The current camera status.
float fpga_power
The current power draw of the FPGA in Watts.
TimeT apply_offset_to_host(const TimeT &input_camera_time) const
Apply the time offset to a camera timestamps to camera timestamp from the reference clock of the came...
bool operator==(const ImageConfig &rhs) const
Equality operator.
TimeT sample_time
The MultiSense timestamp associated with the frame.
std::optional< Measurement > magnetometer
The measured magnetic field in milligauss.
Manual exposure specific configuration.
std::optional< OperatingMode > magnetometer
Configuration for the onboard magnetometer.
TimeT ptp_timestamp
The timestamp associated with the image based using the camera's clock which is potentially PTP synch...
std::array< std::array< float, 3 >, 3 > R
Rotation matrix which takes points in the unrectified camera frame and transform them in to the recti...
std::vector< PcbInfo > pcb_info
Information about each PCB.
uint16_t top_left_x_position
The x value of the top left corner of the ROI in the full resolution image.
DistortionType
Distortion type.
float fpga_temperature
Temperature of the FPGA in Celsius.
uint16_t steps_from_local_to_grandmaster
The number of network hops from the grandmaster to the camera's clock.
Config for a specific IMU operating mode.
uint32_t imager_height
The native height of the primary imager.
std::string name
The name of the IMU sensor.
std::optional< AutoWhiteBalanceConfig > auto_white_balance
The white balance parameters to use if auto white balance is enabled.
std::chrono::microseconds exposure_time
The manual exposure time in microseconds Valid range is [0, 33000].
std::optional< ImuInfo > imu
Supported operating modes for the IMU sensors (accelerometer, gyroscope, magnetometer).
uint16_t height
The height of the ROI in the full resolution image.
std::string build_date
The date the MultiSense was manufactured This value can store at most 32 characters.
@ AUX_CHROMA_RECTIFIED_RAW
std::string lens_name
The name of the lens used for the primary camera For stereo cameras this is the Left/Right stereo pai...
bool processing_pipeline_ok
True if the onboard processing pipeline is ok and currently processing images.
The Device information associated with the MultiSense.
bool operator==(const ImuConfig &rhs) const
Equality operator.
Info for the PCBs contained in the unit.
std::optional< OperatingMode > gyroscope
Configuration for the onboard gyroscope.
std::shared_ptr< const std::vector< uint8_t > > raw_data
A pointer to the raw image data sent from the camera.
SensorVersion version
Sensor Version info.
Stereo specific configuration.
FlashMode
Different flash modes for the camera.
Manual white balance specific configuration.
DataSource source
The camera data source which this image corresponds to.
DeviceInfo device
Device info.
bool operator==(const InternalConfig &rhs) const
Equality operator.
bool ptp_enabled
Enable PTP sync on the camera.
Auto-exposure Region-of-Interest (ROI) specific configuration.
int64_t image_data_offset
An offset into the raw_data pointer where the image data starts.
bool operator==(const ImuRate &rhs) const
Equality operator.
uint32_t height
The operating height of the MultiSense in pixels.
TimeT ptp_sample_time
The MultiSense ptp timestamp associated with the frame.
std::optional< PowerStatus > power
The current power status.
@ PATTERN_PROJECTOR
A pattern projector.
std::optional< AutoExposureConfig > auto_exposure
The exposure config to use if auto exposure is enabled.
bool operator==(const ManualWhiteBalanceConfig &rhs) const
Equality operator.
ImagerType
Different imager types.
float range
The max value the sensor can return.
float y
Measurement on the y-axis of the sensor.
LightingType lighting_type
The type of lighting used in the MultiSense.
bool enabled
Enable the current source.
std::optional< TimeStatus > time
The current timing status information.
bool sharpening_enabled
Enable sharpening.
std::optional< TemperatureStatus > temperature
The current temperature status.
Config for transmitting packets from the MultiSense to the host.
int height
Height of the image in pixels.
bool operator==(const StereoConfig &rhs) const
Equality operator.
std::optional< ExternalConfig > external
The external lighting config.
Version information for the MultiSense.
ClientNetworkStatus client_network
The current client network statistics.
float gamma
Set the gamma correction for the image.
float x
Measurement on the x-axis of the sensor.
@ PATTERN_PROJECTOR_OUTPUT_TRIGGER
A pattern projector with a GPIO line used to trigger a external light.
@ AUX_RECTIFIED_COMPRESSED
bool cameras_ok
True if the cameras are operating and currently streaming data.
StereoCalibration calibration
The scaled calibration for the entire camera.
uint32_t major
Major version number.
std::chrono::nanoseconds grandmaster_offset
Offset between the camera's PTP Hardware Clock and the grandmaster clock.
uint16_t top_left_y_position
The y value of the top left corner of the ROI in the full resolution image.
FlashMode flash
Configure flash mode.
float intensity
Lighting brightness ranging from 0 to 100.0.
uint32_t patch
Patch version number.
TimeT camera_timestamp
The timestamp associated with the image based on the camera's clock.
std::optional< LightingConfig > lighting_config
The lighting configuration for the camera.
LensType
MultiSense lens types.
std::chrono::nanoseconds network_delay
The estimated network delay between when the status request was sent, and when the status request was...
ImuRange range
The specific IMU range configuration specified in ImuInfo::Source.
std::chrono::nanoseconds path_delay
The estimate delay of the PTP synchronization messages from the grandmaster.
int64_t frame_id
The unique monotonically increasing ID for each frame populated by the MultiSense.
uint32_t width
The operating width of the MultiSense in pixels.
Lighting configuration for the camera.
std::map< DataSource, Image > images
The images assocated with each source in the frame.
float z
Measurement on the z-axis of the sensor.
Lighting config for lights driven by GPIO outputs from the MultiSense.
The range for each sensor along with the corresponding sampling resolution.
ImageConfig image_config
Image configuration for the Aux imager.
std::optional< Source > accelerometer
Configuration specific to the accelerometer.
std::chrono::nanoseconds camera_time
The camera's system time when the status message request was received.
TimeT frame_time
The MultiSense timestamp associated with the frame.
std::array< uint8_t, 8 > grandmaster_id
The id of the current grandmaster clock (8 bytes, 0xXXXXXX.XXXX.XXXXXX)
bool grandmaster_present
Status of the grandmaster clock.
size_t dropped_messages
The total number of dropped messages on the client side.
float blue
The manual blue white-balance setting Valid range is [0.25, 4].
MultiSenseConfig::MaxDisparities disparities
Supported operating disparity.
bool operator==(const OperatingMode &rhs) const
Equality operator.
Represents a single image plus metadata.
float postfilter_strength
This is used to filter low confidence stereo data before it is sent to the host.
std::array< std::array< float, 3 >, 3 > K
Unrectified camera projection matrix stored in row-major ordering.
std::array< std::array< float, 4 >, 3 > P
Rectified projection matrix which takes points in the origin camera coordinate frame and projects the...
uint16_t width
The width of the ROI in the full resolution image.
bool operator==(const TimeConfig &rhs) const
Equality operator.
MULTISENSE_API std::string to_string(const Status &status)
Convert a status object to a user readable string.
std::optional< PtpStatus > ptp
The current ptp status.
std::optional< InternalConfig > internal
The internal lighting config.
size_t image_data_length
The length of the image data after the image_data_offset has been applied.
uint32_t minor
Minor version number.
std::optional< Measurement > accelerometer
The acceleration in units of Gs.
float left_imager_temperature
Temperature of the left imager in Celsius.
Information about the IMU onboard the MultiSense.
bool operator==(const LightingConfig &rhs) const
Equality operator.
Complete configuration object for configuring the MultiSense.
size_t invalid_packets
The total number of invalid packets received on the client side.
float target_threshold
The fraction of pixels which must be equal or below the pixel value set by the target intensity pixel...
bool has_image(const DataSource &source) const
Check if we have an image for a given data source.
bool system_ok
Summary of the current MultiSense state.
bool operator==(const ManualExposureConfig &rhs) const
Equality operator.
@ LEFT_RECTIFIED_COMPRESSED
uint32_t samples_per_frame
The number of IMU samples which should be included in a IMU frame.
bool operator==(const AuxConfig &rhs) const
Equality operator.